How to refresh data in Nuxt 3 using the watch option
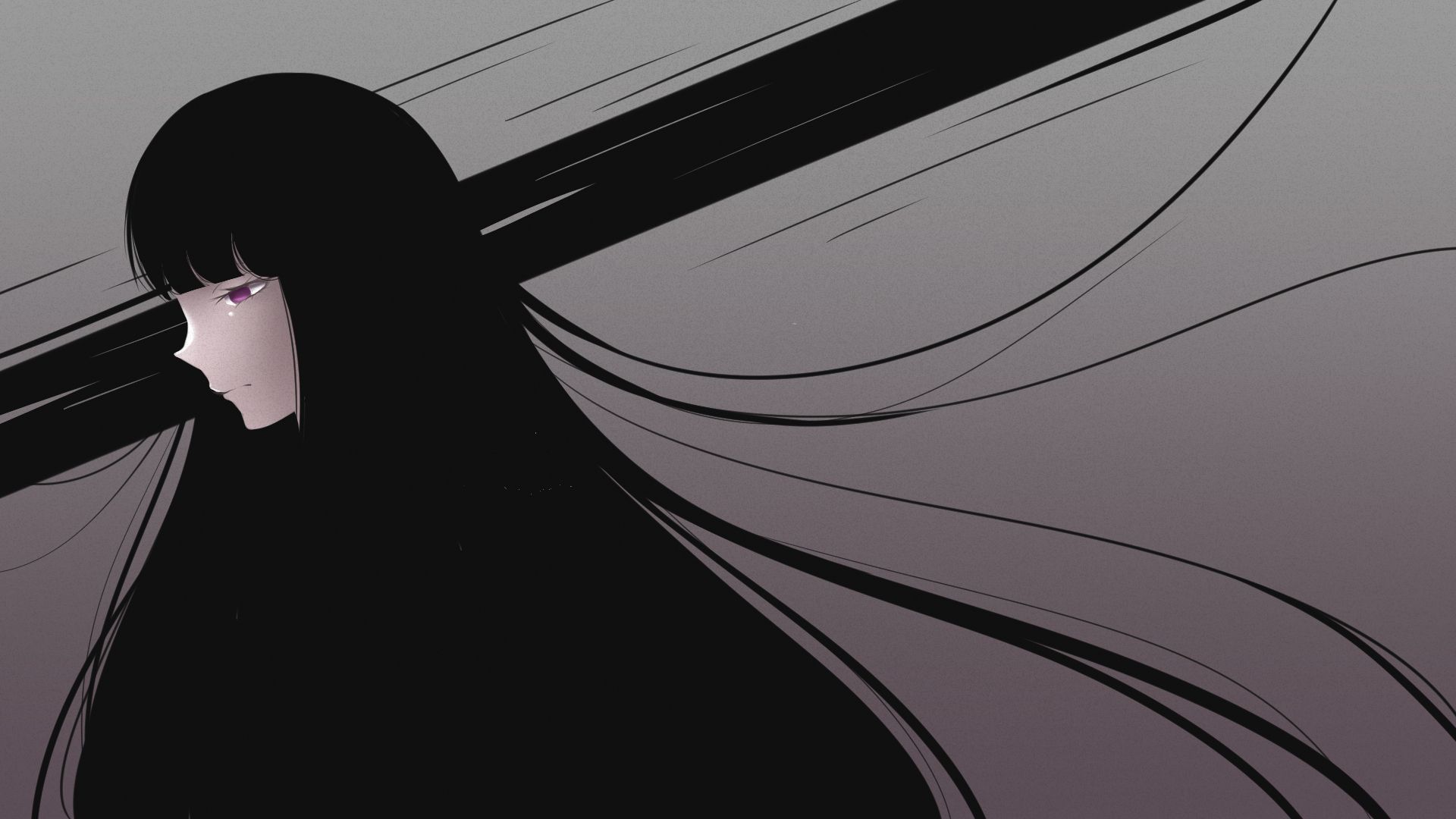
At one moment, if you have been using useFetch
, useLazyFetch
, useAsyncData
and useLazyAsyncData
to handle data fetching within your application, you may need to refresh the data loaded from the API based on the user's action. (pagination, filter ing results, searching, etc.)
You can use the refresh()
method returned from the useFetch
, useLazyFetch
, useAsyncData
and useLazyAsyncData
composable to refresh the data with different parameters:
// Code copied and adapted from the official documentation
<script setup>
const page = ref(1);
const { data: users, pending, refresh, error } = await useFetch(`users?page=${page.value}&take=6`, { baseURL: config.API_BASE_URL });
// const { data: users, pending, refresh, error } = await useAsyncData("users", () => $fetch(`${config.API_BASE_URL}users?page=${page.value}&take=6`), {});
function previous(){
page.value--;
refresh();
}
function next() {
page.value++;
refresh();
}
</script>
Another possibility that I prefer is the usage of the watch option with useFetch
, useLazyFetch
, useAsyncData
and useLazyAsyncData
composable.
Providing this option will watch all reactive sources (in our case page
) to auto-refresh. The updated code will look like this:
// Code copied and adapted from the official documentation
<script setup>
const page = ref(1);
const { data: users, pending, error } = await useFetch(`users?page=${page.value}&take=6`, { baseURL: config.API_BASE_URL, watch:[page] });
// const { data: users, pending, error } = await useAsyncData("users", () => $fetch(`${config.API_BASE_URL}users?page=${page.value}&take=6`), { watch:[page] });
function previous(){
page.value--;
}
function next() {
page.value++;
}
</script>
Conclusion
The watch option is nice feature to make your code more reactive!
Of course, if you have any questions, feel free to reach out!